XSS
Let us delve into the concept of the Same Origin Policy (SOP), a fundamental security measure in web browsing. The SOP serves as a safeguard against unauthorized access and data manipulation between different websites. It operates by thoroughly scrutinizing three distinct origin components, including the protocol, host, and port. Only if all three parameters match for two distinct origins, will the browser permit cross-origin read or write actions.
For instance, consider the following scenario involving two websites, https://example.com and https://hack.com. In this case, the browser will first verify if the protocol is the same for both sites. Since they both use HTTPS, the protocol matches. However, the browser will subsequently identify that the host (example.com vs. hack.com) is different and therefore, restrict any cross-origin read or write actions.
In essence, SOP restricts HTTP requests that one origin can send to another. It only permits cross-origin HTTP requests within the same domain (host), and only GET and POST methods are allowed, while PUT and DELETE requests are denied.Nevertheless, there is an exception to SOP known as Cross-Origin Resource Sharing (CORS). This mechanism enables SOP bypass, but only with the explicit consent of the server. This feature is quite useful as APIs are ubiquitous, and usually, APIs are hosted on a different domain from the one that makes requests. In such situations, it becomes necessary to establish a proper way to communicate between different domains.
Finally, it is important to note that there may be confusion surrounding SOP in terms of Cross-Site Request Forgery (CSRF). In CSRF attacks, there is no SOP bypass. The attacker is essentially making requests to other websites, which are allowed. However, they cannot read the responses due to SOP restrictions, which are not violated.
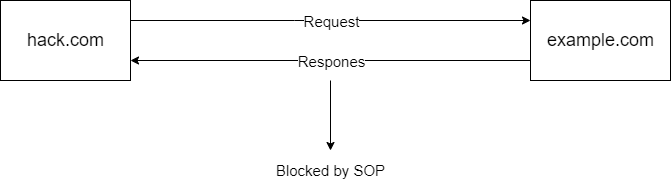
So SOP is a great feature insuring some basic web security.
As we all know, JavaScript has access to HTML documents via the Document Object Model (DOM) APIs, which are already provided by the browser. This grants us the ability to manipulate the DOM and alter the appearance of a webpage or even steal some CSRF tokens, which can be quite problematic. In some cases, we can even read cookies and send them to our website through AJAX requests or form submissions.
Having control over JavaScript on another website in a different context can pose a significant threat. This begs the question: "Can we inject JS into another website?" The answer is a resounding "YES." This is precisely what Cross-Site Scripting (XSS) is all about: a JavaScript injection technique that can be used to take advantage of vulnerabilities in a website's input validation mechanisms.
In essence, XSS attacks can enable an attacker to inject malicious code into a webpage, which can subsequently be executed by unsuspecting users who visit the site. This can allow the attacker to steal sensitive user information, such as login credentials, financial data, or personal information.
Therefore, it is crucial to be aware of XSS vulnerabilities and implement appropriate security measures to prevent such attacks. These measures may include input validation, sanitization of user input, and the use of Content Security Policies (CSP) to restrict the execution of external scripts on a website.
Now lets look at some example:
Suppose there's a website where we have to enter our name, so we simply just write our name and submit as usual. And on clicking submit button we send out an http request.
Which would look something like this:
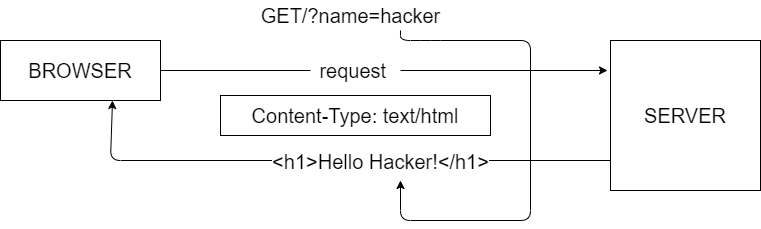
Now, the interesting fact is that the browser perceives the entire response as HTML, as denoted by the content-type header specified as text/html.
Lets repeat the same request but this time, lets use something which looks like this:
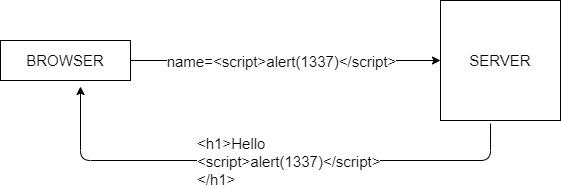
A similar thing will happen: we get back the input directly in the response but as you can see there is no difference between the code that needs to be rendered and the input we send out. So it simply accepts the whole thing as html and executes our Javascript. This is called XSS.
Here, in this Javascript code website is vurnerable to XSS so we get a pop-up of 1337.
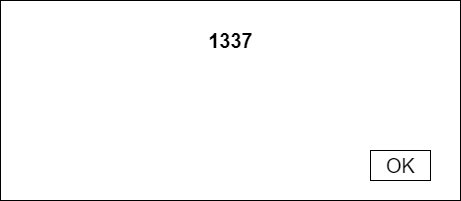
This above example was of reflected XSS.
Stored XSS
It's similar to reflected XSS, but the input isn't simply reflected back. Instead, the input is persisted, meaning it's stored in some sort of database or other location, and then displayed to the user by retrieving it from where it was stored.
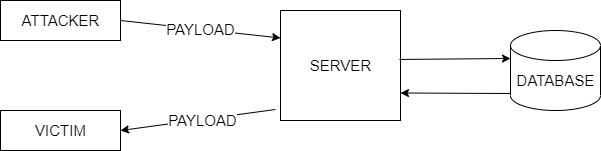
However, this type of attack can be even more powerful as the malicious input is stored in a database and can infect anyone who views a page that relies on that input. One example is the comment section of YouTube, where anyone who simply views the comments can become infected without realizing it.
DOM XSS
Its a vulnerability where user's input directly affects the inner dangerous part of the JS code and this entirely happens on clients side.
For example:
The user input directly affects the inner html property of the div element. So in this case a string supply by the user affects inside a DOM which ultimately has ability to execute some JS.
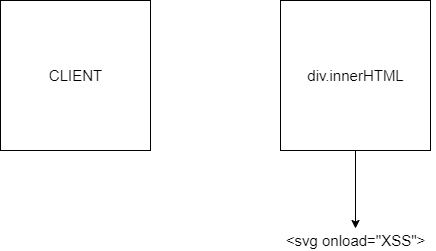
M XSS or Mutation XSS
Its a vulnerability where the user's input is mutated or changed in someway by the browser before inserting it to the DOM which sometimes leads to XSS.

PROBLEM
Blocking script tag might be fix of XSS but that wont work entirely.
Script tags aren't the only way to execute JavaScript; you can also use event handlers that are associated with most HTML tags to execute JavaScript code.
Examples of Event Handlers:

SOLUTION
To prevent XSS attacks, it's important to filter input upon arrival, encode data on output, use appropriate response headers, and, as a last resort, use a Content Security Policy to reduce the severity of any XSS vulnerabilities that may occur.